本文指导您在OpenAPI门户自动生成对应接口的SDK示例。
示例场景
本文以在OpenAPI门户调用云服务器DescribeAvailableResource,查询您在某一可用区的资源列表为例,自动生成Java SDK示例代码。
操作步骤
一、快速下载工程
适用于初次接触阿里云SDK的用户,通过以下步骤您可以获取完整示例工程代码,工程示例包含完整依赖信息,入参,请求,打印响应等。
单击OpenAPI门户。
参考图中示例步骤获取SDK工程示例代码。
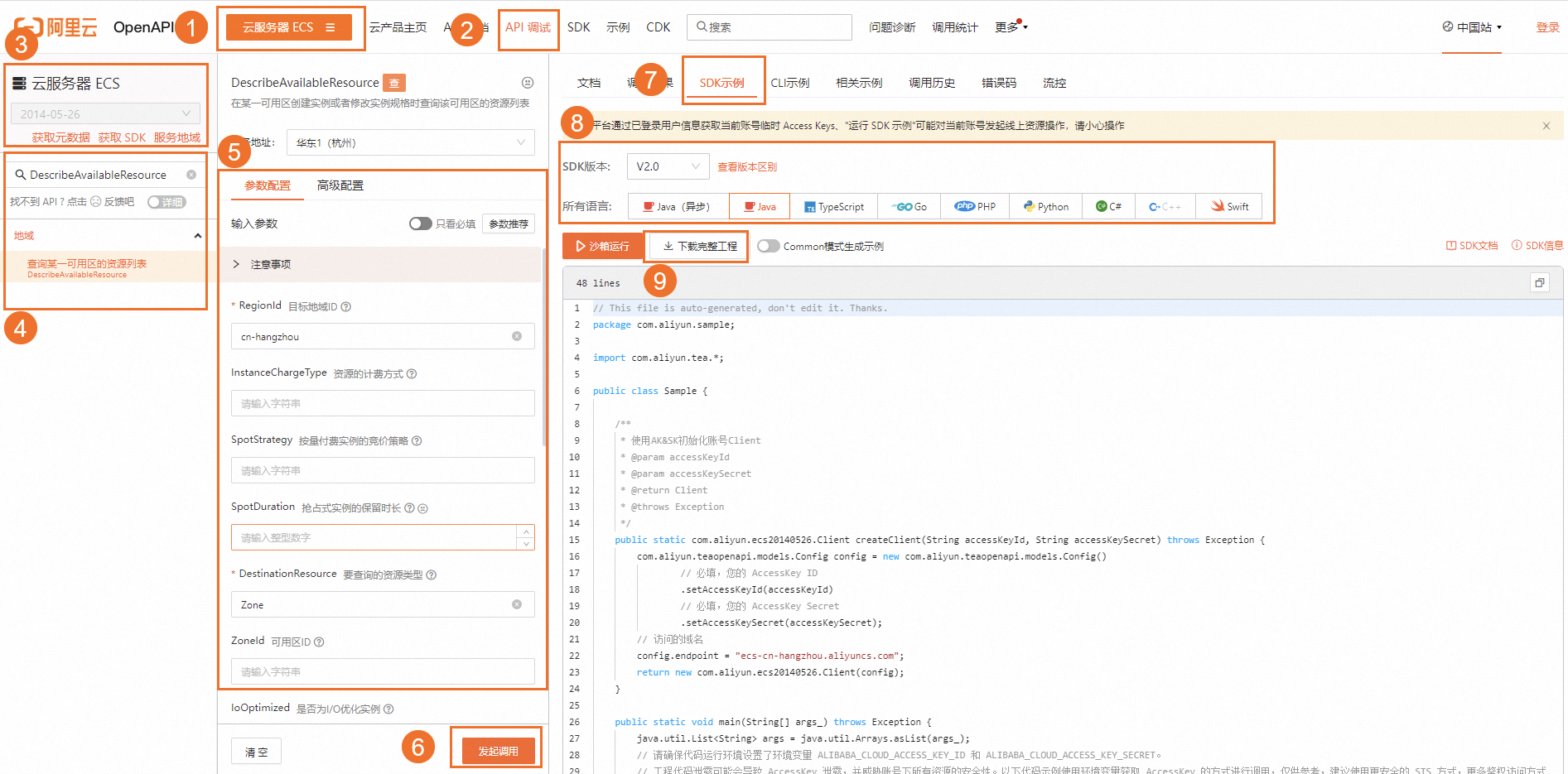
说明如下:
序号 | 相关操作 |
① | 在OpenAPI 门户页面顶部,单击图标 |
② | 在OpenAPI 门户页面顶部,单击API 调试。 |
③ | API版本。例如ecs |
④ | 在搜索框中输入目标API接口,然后在API接口显示后单击API接口。 例如输入 |
⑤ | 配置 |
⑥ | (非必要步骤)发起正式调用,若是付费接口将可能产生费用。 |
⑦ | 在页面右侧区域的顶部,单击SDK示例。 |
⑧ | 按需选择SDK版本和语言。 例如SDK版本选择 |
⑨ | 下载完整示例工程代码,包含打印逻辑及依赖信息。 |
二、复制示例代码和SDK信息
适合已经比较熟悉阿里云SDK的开发者用户,可快速获取关键代码逻辑,示例代码不打印响应和异常。
单击OpenAPI门户。
参考图中示例步骤获取SDK信息和示例代码。
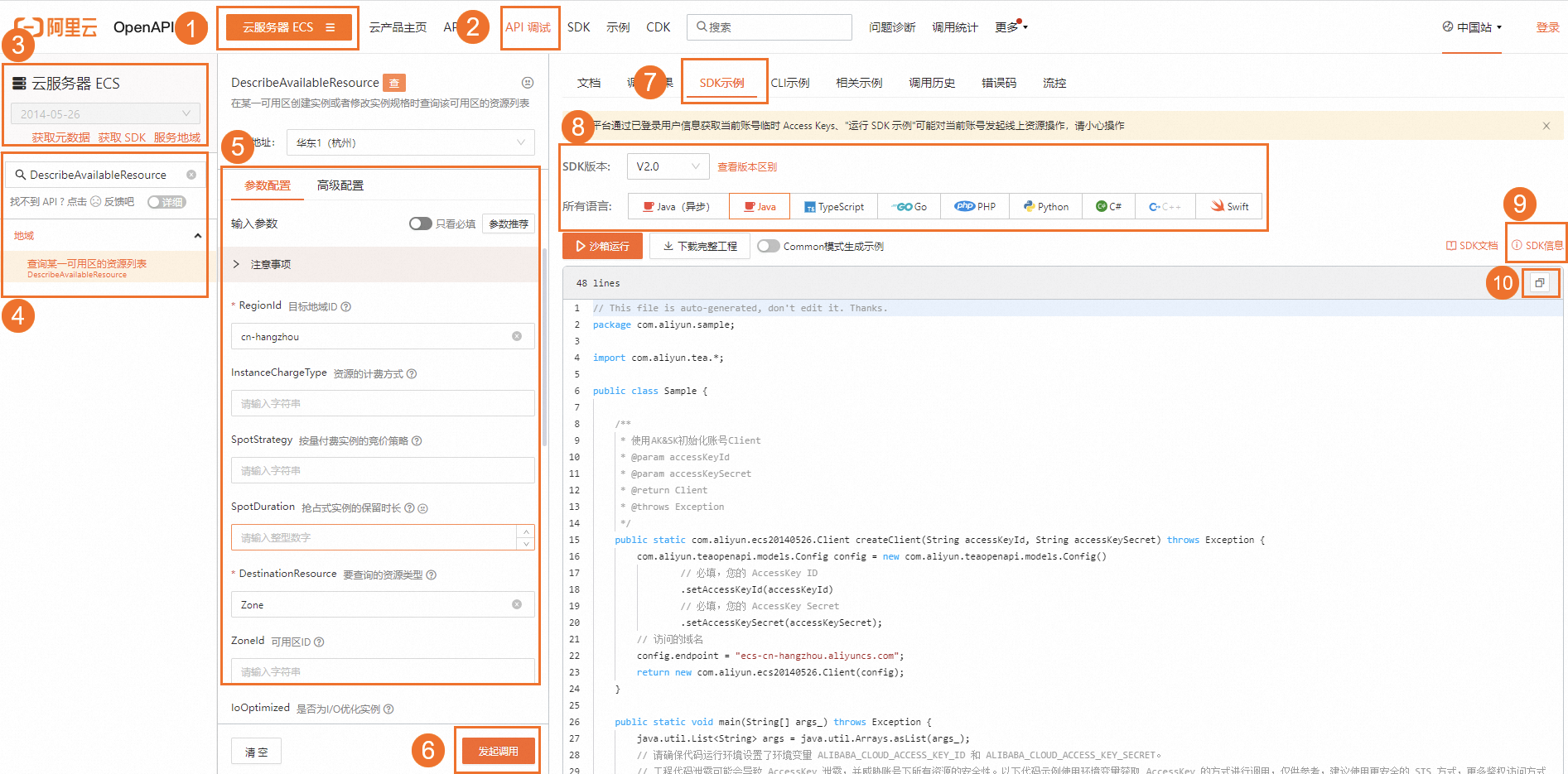
说明如下:
序号 | 相关操作 |
① | 在OpenAPI 门户页面顶部,单击图标 |
② | 在在OpenAPI 门户页面顶部,单击API 调试。 |
③ | API版本。例如ecs |
④ | 在搜索框中输入目标API接口,然后在API接口显示后单击API接口。 例如输入 |
⑤ | 配置 |
⑥ | (非必要步骤)发起正式调用,若是付费接口将可能产生费用。 |
⑦ | 在页面右侧区域的顶部,单击SDK示例。 |
⑧ | 按需选择SDK版本和语言。 例如SDK版本选择 |
⑨ | 查看SDK信息,获取依赖安装命令和发布报告。 |
⑩ | 复制页面生成的代码示例接入,该示例不包含打印逻辑。 |
在SDK示例显示区域的右上角,单击
图标可以复制SDK示例代码。
在SDK示例显示区域的顶部,单击下载完整工程可以下载完整的SDK工程。
- 本页导读 (1)