概览
介绍Assistant API的使用方法。
1. Assistant API简介
百炼Assistant API能够让用户定制化构建一个assistant,这个assistant支持多种不同的指令(instruction)和描述(prompt),并且可以使用各类工具插件(plugins)和调用已选择的API来回答用户的问题。
目前支持多种插件,如代码解释器、图片生成、夸克搜索等,也支持function-call和自定义插件的录入。
2. 创建和使用Assistant API
与OpenAI类似,我们支持开发者通过代码的形式创建Assistant,目前已支持通过Python代码进行创建(Java版本即将上线),并且我们的代码框架基本与OpenAI完全一致,便于开发者进行代码的迁移和部署。
通常通过代码创建和使用一个Assistant需要以下几个步骤:
1、准备好开发环境,如Python IDE 并安装dependency,并设置百炼用户密钥(api_key);
2、定义开发者所需要的Assistant的指令(instruction)和描述(prompt),并选择需要的模型和涵盖的插件,API,函数(Function)。
3、创建一个智能体(Assistant),作为存储用户配置的实体;
4、创建一个线程(Thread),用于用户开启一段对话;
5、增加一个或多个信息(Message)进线程(Thread),作为用户的提问(Query);
6、运行(Run)Assistant在这些线程(Thread)上,让Assistant生成回答,并智能调用一个或多个插件/函数下面给出一个具体的流程示例,开发者可以通过下述代码进行快速尝试,并创建一个自己的assistant-api使用。
每个步骤的代码示例如下。
Step.1 准备开发环境
目前Assistant API支持Python 3.8及以上版本,首先安装相关依赖包。
pip install dashscope>=1.17.0
安装后,在代码中需要传入对应的用户在百炼的密钥,用于开启大模型的使用。一种简单的方式是通过定义全局变量,即如下所示。
设置您的API-KEY,替换YOUR_DASHCOPE_API_KEY为您自己的API key
export DASHSCOPE_API_KEY=YOUR_DASHSCOPE_API_KEY
Step.1 创建Assistant
通过Dashscope.Assistant类的create可以完成创建。下面演示了一个Assistant创建的例子,使用图像生成插件。其中verify_status_code函数用于校验是否创建完成。
from http import HTTPStatus
import dashscope
# create assistant with instructions
assistant = dashscope.Assistants.create(
model='qwen-max',
name='My helper',
description='A tool helper.',
instructions='You are a helpful assistant. When asked a question, use tools wherever possible.',
tools=[{ # tool to use, current we support text to image wanx, quark_search, and function call.
'type': 'wanx'
}, {
'type': 'quark_search'
}, {
'type': 'function',
'function': {
'name': 'big_add',
'description': 'Add to number',
'parameters': {
'type': 'object',
'properties': {
'left': {
'type': 'integer',
'description': 'The left operator'
},
'right': {
'type': 'integer',
'description': 'The right operator.'
}
},
'required': ['left', 'right']
}
}
}],
)
# check result is success.
if assistant.status_code != HTTPStatus.OK:
print('Create Assistant failed, status code: %s, code: %s, message: %s' % (assistant.status_code, assistant.code, assistant.message))
else:
print('Create Assistant success, id: %s' % assistant.id)
Step.2 创建Thread
Thread是一个连接开发者和1个或多个Assistant的桥梁。
创建一个Thread,用于传入后续一个或多个message,用于得到assistant请求。
from http import HTTPStatus
import dashscope
thread = dashscope.Threads.create(messages=[{"role": "user", "content": "Tell me something about AI."}])
# check result is success.
if thread.status_code != HTTPStatus.OK:
print('Create Thread failed, status code: %s, code: %s, message: %s' % (thread.status_code, thread.code, thread.message))
else:
print('Create Thread success, id: %s' % thread.id)
Step.3 为Thread创建Message
用户需要query的信息可以通过Message传入,可以创建一个或多个Message给线程(Thread);
在创建Message时需要指定待传入的thread_id;
传入一个Thread的token长度没有限制,但是真正传入大模型的token长度受限于大模型的最大可支持长度。通义千问系列模型支持的上下文长度见模型介绍。
下面以“画一个可爱的猫为例”,创建一个Message类并指定Thread的id。
from http import HTTPStatus
import dashscope
# Create a message, tell the Assistant what needs to be done?
message = dashscope.Messages.create('the_thread_id', content='Draw a picture of a cute kitten lying on the sofa.')
if message.status_code != HTTPStatus.OK:
print('Create Message failed, status code: %s, code: %s, message: %s' % (message.status_code, message.code, message.message))
else:
print('Create Message success, id: %s' % message.id)
Step.5 Run Assistant中的Thread
当用户将Message已经指定到某一个具体的Thread后,用户可以通过“Run”这个Thread来运行创建好的Assistant。
创建“Run”会让Assistant通过指定的模型和插件候选集,智能地选择并回答用户的问题。
最终生成的答案也会添加进Thread的Message里。
注意:
由于可能模型使用人数过多,可能需要进行一定时间的等待运行结束,因此建议等待Run的状态为“complete”后继续运行。
下面的例子给了一个提交run并等待取回最终response的示例:
from http import HTTPStatus
import json
import dashscope
# create a new run to run message
run = dashscope.Runs.create('your_thread_id', assistant_id='your_assistant_assistant_id')
if run.status_code != HTTPStatus.OK:
print('Create Assistant failed, status code: %s, code: %s, message: %s' % (run.status_code, run.code, run.message))
else:
print('Create Assistant success, id: %s' % run.id)
# wait for run completed or requires_action
run = dashscope.Runs.wait(run.id, thread_id='your_thread_id')
if run.status_code != HTTPStatus.OK:
print('Get run failed, status code: %s, code: %s, message: %s' % (run.status_code, run.code, run.message))
else:
print(run)
# get the thread messages, to get the run output.
msgs = dashscope.Messages.list('your_thread_id')
if msgs.status_code != HTTPStatus.OK:
print('Get msgs failed, status code: %s, code: %s, message: %s' % (msgs.status_code, msgs.code, msgs.message))
else:
print(json.dumps(msgs, default=lambda o: o.__dict__, sort_keys=True, indent=4))
最终结果如下,在‘text'字段的’value'中是assistant-api生成的答案,“Here's the AI-generated image..”
{'has_more': False, 'last_id': 'message_7788fec4-22d1-483d-949f-5aa7c2b7b2d8', 'first_id': 'message_f88ad175-24b3-493a-b692-97312bb91608', 'data': [{'id': 'message_f88ad175-24b3-493a-b692-97312bb91608', 'created_at': 1710839845513, 'thread_id': 'thread_c14c9ca6-200b-49e3-bb5d-879e731f7d9b', 'role': 'assistant', 'content': [{'text': {'value': "Here's the AI-generated image based on your prompt:\n\n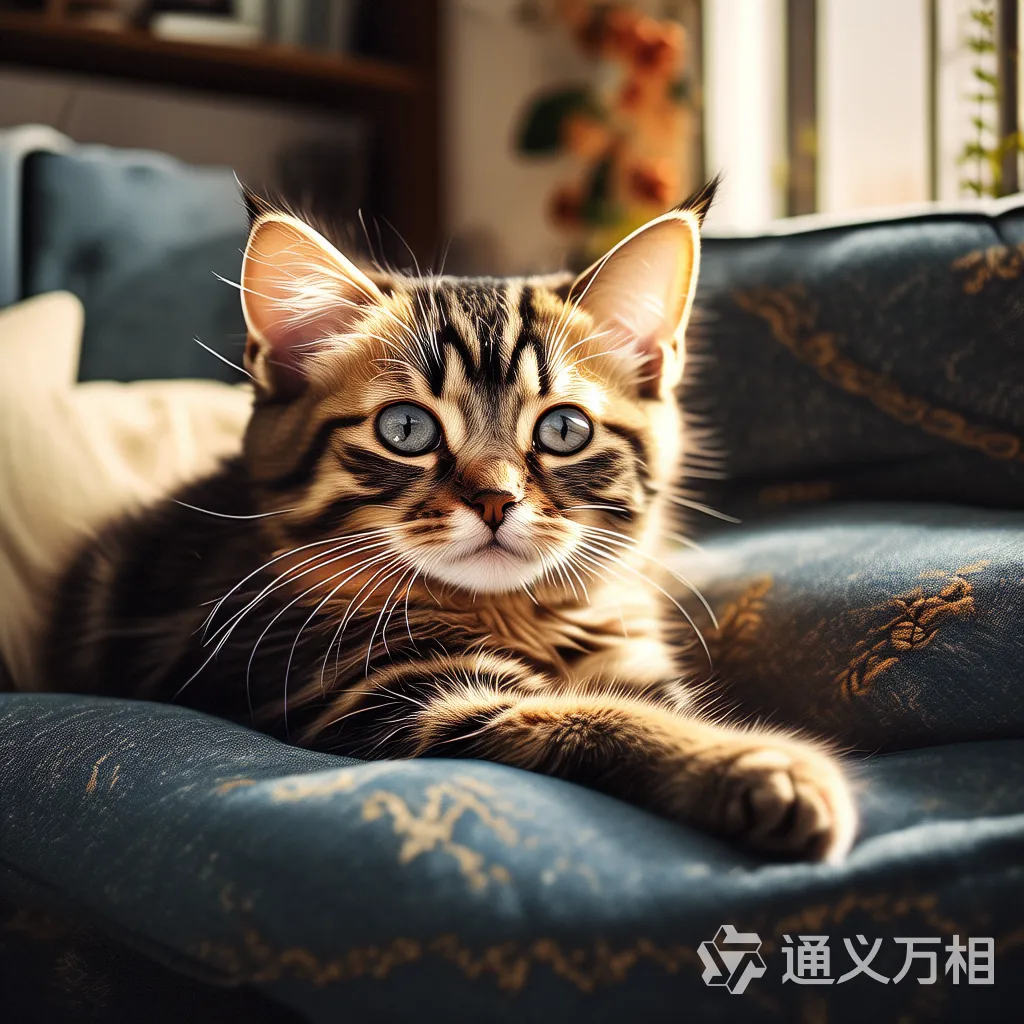"}, 'type': 'text'}], 'metadata': {}, 'object': 'thread.message', 'assistant_id': 'asst_c5bc5fd5-940f-4391-aa00-f5ca8a1b0e2f', 'file_ids': [], 'run_id': 'run_fbd195eb-84a9-4e60-b2b4-cb06cf9e1820'}, {'id': 'message_7788fec4-22d1-483d-949f-5aa7c2b7b2d8', 'created_at': 1710839804254, 'thread_id': 'thread_c14c9ca6-200b-49e3-bb5d-879e731f7d9b', 'role': 'user', 'content': [{'text': {'value': 'Draw a picture of a cute kitten lying on the sofa.'}, 'type': 'text'}], 'metadata': {}, 'object': 'thread.message', 'assistant_id': '', 'file_ids': [], 'run_id': ''}], 'object': 'list', 'request_id': '76b2a584-caf8-9cf2-b0b3-dc8c7864f6d4', 'status_code': 200}
3. 官方大语言模型
通义千问-Max(qwen-max)
目前Assistant API仅支持千问系列模型qwen-max。模型的具体信息可以参考模型介绍。
其他大模型
其他大模型及用户自训练的大模型计划近期支持。
4. 官方内置插件
Python代码解释器(Code Interpreter)
通过代码方式使用该插件的方式如下,即在tools添加“code_interpreter”即可。
import dashscope
assistant = dashscope.Assistants.create(model='qwen-max',
name='smart helper',
description='A tool helper.',
instructions='You are a helpful assistant. When asked a question, use tools wherever possible.',
tools=[{
'type': 'code_interpreter'
}, ])
图片生成(Wanx)
通义万相是一款基于文生图的应用,目前已集成进阿里云百炼Assistant API的官方插件,名称为图片生成。
通过代码方式使用该插件的方式如下,即在tools添加“wanx”即可。
import dashscope
assistant = dashscope.Assistants.create(model='qwen-max',
name='smart helper',
description='A tool helper.',
instructions='You are a helpful assistant. When asked a question, use tools wherever possible.',
tools=[{
'type': 'wanx'
}, {
'type': "code_interpreter"
}],)
夸克搜索(quark_search)
百炼提供了夸克搜索插件,支持用户通过Assistant API搜索实时结果。
调用方式是在tools里添加“quark_search",即可使assistant在有需要的时候智能调用该插件。
import dashscope
assistant = dashscope.Assistants.create(model='qwen-max',
name='smart helper',
description='A tool helper.',
instructions='You are a helpful assistant. When asked a question, use tools wherever possible.',
tools=[{
'type': 'quark_search'
}, {
'type': "code_interpreter"
}])
Function Calling
除了上述插件,Assistant API也支持Function Calling的功能。对于部分插件或计算,为了满足本地计算等需求,可以通过Function Calling 实现。
用户描述可能被调用的一个或多个函数和它们的输入输出参数。
当通过Run运行Assistant时,若调用到所需要的外部函数,系统会暂停并进入“require_action”状态,返回具体调用的函数名和输入参数。用户需要自行在外部环境执行完Assistant所选择的函数(使用生成的入参),并将函数结果通过“submit_tools_by_run”返回给Assistant。随后,Run的状态会继续进入运行状态直至“complete”。
下面是大数计算的一个具体例子。
import dashscope
assistant = dashscope.Assistants.create(model='qwen-max',
name='smart helper',
description='A tool helper.',
instructions='You are a helpful assistant. When asked a question, use tools wherever possible.',
tools=[{
'type': 'wanx'
}, {
'type': 'function',
'function': {
'name': 'big_add',
'description': 'Add to number',
'parameters': {
'type': 'object',
'properties': {
'left': {
'type': 'integer',
'description': 'The left operator'
},
'right': {
'type': 'integer',
'description': 'The right operator.'
}
},
'required': ['left', 'right']
}
}
}],)
随后构建thread和message,并通过“submit_tool_outputs” 传入结果。
import dashscope
# create thread.
thread = dashscope.Threads.create()
# create a message.
message = dashscope.Messages.create(thread.id, content='Add 87787 to 788988737.')
message_run = dashscope.Runs.create(thread.id, assistant_id=assistant.id)
# get run statue
run_status = dashscope.Runs.get(message_run.id, thread_id=thread.id)
# if prompt input tool result, submit tool result.
# should call big_add
if run_status.required_action:
tool_outputs = [{
'tool_call_id':
run_status.required_action.submit_tool_outputs.tool_calls[0].id,
'output':
'789076524'
}]
run = dashscope.Runs.submit_tool_outputs(message_run.id,
thread_id=thread.id,
tool_outputs=tool_outputs)
print(run)
# wait for run completed or requires_action
run_status = dashscope.Runs.wait(message_run.id, thread_id=thread.id)
# get the thread messages.
msgs = dashscope.Messages.list(thread.id)
print(msgs)
自定义插件
用户可以通过百炼插件中心注册自定义插件,注册成功后,系统会生成一个全局唯一的插件ID。获取到该插件ID后,您可以将其放入tools中的type字段,即可正常调用该插件。
- 本页导读 (1)